Modern JavaScript Compilers: TypeScript and Babel
- SquareShift Engineering Team
- Dec 13, 2024
- 3 min read
Compilers are essential tools in modern software development, transforming source code into executable programs. In JavaScript, compilers play a crucial role in optimizing performance and ensuring cross-platform compatibility. Initially focused on basic transformations, compilers have evolved to support advanced features like ES6+ syntax and TypeScript, enabling developers to write cleaner, more efficient code.

TypeScript
TypeScript is a statically typed superset of JavaScript that introduces optional static typing, interfaces, generics, and type aliases. These features enable developers to define types, catch errors at compile-time, and reduce runtime issues, improving code quality. TypeScript is fully compatible with JavaScript, making it easy to migrate existing projects incrementally. Additionally, it offers robust tooling such as enhanced autocompletion, refactoring, and debugging to boost developer productivity.

Use Cases:
Large-Scale Web Applications: TypeScript’s static typing improves the scalability and maintainability of large applications by catching errors at compile-time, helping to save time and reduce bugs.
Example: Airbnb uses TypeScript to manage its complex web architecture, improving code quality and scalability.
Enterprise-Grade Applications: With TypeScript’s strong typing, large teams can collaborate more easily, ensuring better code quality and reliability for enterprise systems.
Example: Microsoft uses TypeScript for products like Azure, benefiting from enhanced code safety and IDE support.
Front-End Development with Frameworks: TypeScript integrates seamlessly with modern front-end frameworks like React, Angular, and Vue, reducing runtime errors and enhancing code scalability.
Example: Slack uses TypeScript for its front-end to ensure smooth, scalable updates.
Server-Side Development: TypeScript can be used in Node.js for full-stack development, ensuring consistency and minimizing errors between client and server code.
Example: LinkedIn uses TypeScript in its Node.js server-side code, streamlining development and improving productivity.
Mobile App Development: With React Native, TypeScript enhances cross-platform mobile app performance by catching errors before they occur at runtime.
Example: Walmart uses TypeScript in their mobile apps built with React Native, improving performance and maintainability.
Compile TypeScript to JavaScript:
tsc <filename>.ts
Performance: TypeScript’s efficient compiler generates optimized JavaScript, preventing runtime bugs through its static type checking.
Babel
Babel is a powerful JavaScript compiler widely used to take advantage of the latest ECMAScript features (ES6 and beyond) while ensuring compatibility with older browsers and environments. By transpiling modern JavaScript syntax into widely-supported versions, Babel allows developers to utilize cutting-edge language features without worrying about compatibility issues.
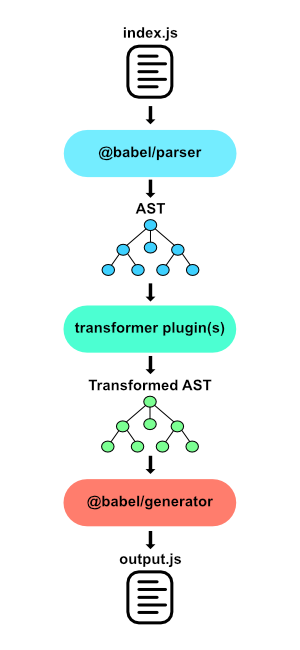
Use Cases:
Transpiling Modern JavaScript for Browser Compatibility: Babel enables developers to write modern JavaScript (ES6+) while ensuring it works across older browsers by converting the code to ES5.
Example: Netflix uses Babel to ensure their web application works seamlessly across various browsers, including older versions.
Support for JSX and React Development: Babel is crucial in React projects for transpiling JSX into JavaScript, allowing developers to use React’s component-based structure without browser compatibility issues.
Example: Facebook uses Babel to ensure JSX code runs smoothly across all browsers in their React applications.
Polyfilling New JavaScript Features: Babel can automatically include necessary polyfills to ensure new language features, such as async/await, work in older environments.
Example: Airbnb uses Babel to ensure new JavaScript features are available across various browsers by including appropriate polyfills.
Customization of JavaScript Syntax with Plugins: Babel supports a wide range of plugins that allow developers to customize their syntax, such as enabling class properties, decorators, or private methods.
Example: Twitter uses Babel plugins to experiment with advanced JavaScript features in their front-end.
Optimizing Code for Production: Babel can be used to minify and optimize JavaScript code, reducing file size and improving application performance.
Example: Instagram uses Babel to optimize and minimize their JavaScript code for faster load times and a better user experience.
Transpile JavaScript with Babel:
npx babel <filename> --out-file <outputfile>.js
Performance: Although Babel introduces some performance overhead due to its transformation process, its flexibility compensates for this in most projects.
Conclusion:
TypeScript and Babel play complementary roles in modern JavaScript development. TypeScript improves scalability and maintainability with static typing and advanced tooling, while Babel ensures compatibility with the latest JavaScript features across environments. Together, they enable developers to build high-performance, cross-platform applications.
We will explore Flow and PureScript, two tools that optimize performance and enhance code quality in JavaScript development.
Comments