Modern JavaScript Compilers: Flow and PureScript
- SquareShift Engineering Team
- Dec 13, 2024
- 4 min read
Compilers play a crucial role in modern software development, converting source code into optimized executable programs. With JavaScript's continuous evolution, developers rely on advanced tools to enhance code efficiency, maintainability, and compatibility. In the previous blog, we delved into TypeScript and Babel—two popular compilers that empower developers to build robust applications. In this post, we will focus on Flow and PureScript, two sophisticated tools that bring unique strengths to JavaScript development, offering advanced type safety, improved code quality, and scalability
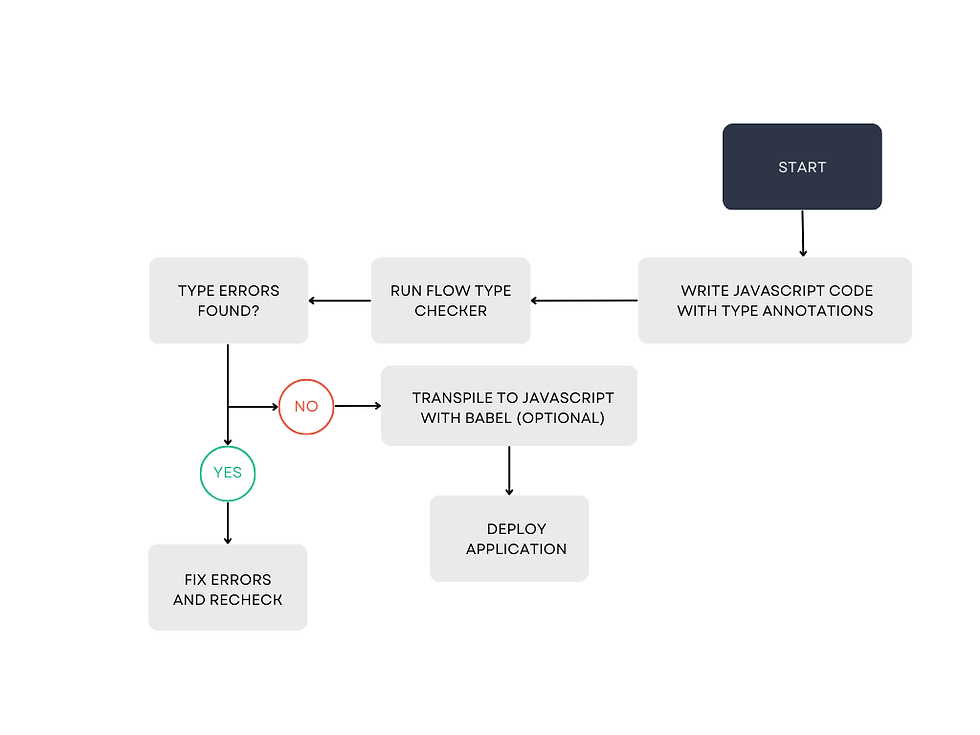
Flow
Flow is a static type checker for JavaScript, developed by Facebook. While not a full-fledged compiler, Flow integrates into your JavaScript codebase and provides static type checking to catch potential errors at compile-time. Unlike TypeScript, which is a superset of JavaScript, Flow operates as an optional type system for JavaScript, allowing you to gradually adopt type checking in your project without requiring a complete rewrite.
Use Cases:
Gradual Type Safety: Flow is ideal for teams that want to introduce type checking into their JavaScript projects without committing to a new language or full rewrite. It’s especially useful for large, legacy codebases where adopting TypeScript would be too disruptive.
Example: Facebook uses Flow to improve the reliability of their JavaScript code without requiring a full migration to TypeScript.
Error Prevention in Large Codebases: Flow’s type checking can significantly reduce the likelihood of bugs in large codebases, where runtime errors are harder to catch. By catching type errors early, Flow can improve code quality and productivity.
Example: Airbnb uses Flow to catch errors in their JavaScript codebase, enhancing collaboration between developers and preventing costly bugs.
Dynamic Type Checking: Flow allows for dynamic typing in certain parts of your application, making it more flexible than TypeScript for some use cases while still providing valuable type safety.
Example: In complex, rapidly-changing applications, Flow’s ability to gradually apply type annotations without disrupting the workflow can be beneficial.
How to Use Flow:
flow init
flow check <filename>.js
Metrics:
Adoption: Flow is widely adopted by companies with large JavaScript codebases that prefer incremental typing.
GitHub Stars: 22.5k+
Community: While the project’s active development has slowed, it remains a popular tool among teams already using it for gradual adoption of type checking.
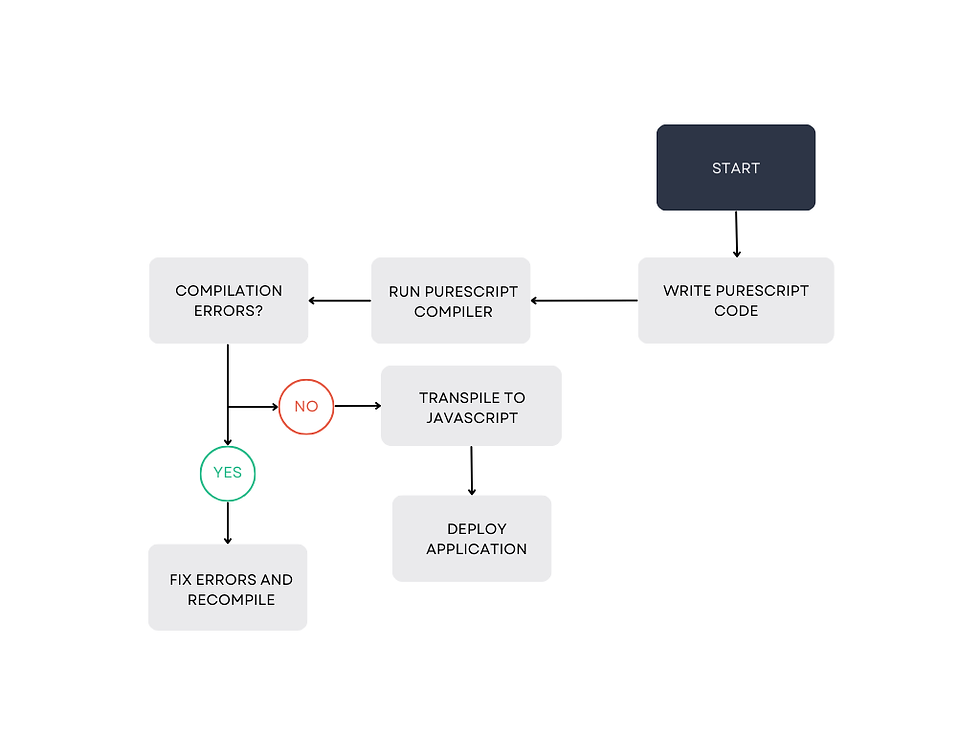
PureScript
PureScript is a strongly-typed, functional programming language that compiles to JavaScript. While it’s not a JavaScript superset like TypeScript, it offers a completely different approach to writing JavaScript code.
Use Cases:
Functional Programming Enthusiasts: Developers familiar with or interested in functional programming will find PureScript a powerful tool for building robust, maintainable JavaScript code. Its functional programming features make it ideal for applications that require highly predictable code and minimal side effects.
Example: Projects that require complex state management or mathematical computations can benefit from PureScript's immutability and strong typing.
Large-Scale Projects: The strong typing system in PureScript ensures that developers can catch errors early in the development cycle. This makes it a great choice for large, complex projects that require strong guarantees of correctness and maintainability.
Example: Financial applications or other mission-critical systems where type safety is paramount can benefit from PureScript.
Interoperability with Existing JavaScript Projects: PureScript allows developers to integrate with existing JavaScript codebases, enabling the adoption of functional programming in parts of a project without having to rewrite everything.
Example: Large web applications that need a functional approach to certain modules or components can benefit from PureScript’s ability to seamlessly integrate with JavaScript.
The below is the code which can be compiled using PureScript compiler
main :: Effect Unit
main =
render $ fold
[ h1 (text "Try PureScript!")
, p (text "Try out the examples below, or create your own!")
, h2 (text "Examples")
, list (map fromExample examples)
, h2 (text "Share Your Code")
, p (text "The contents of the text editor are stored in the URL. To share your code you can simply copy the URL.")
, p (text "Additionally, a PureScript file can be loaded from GitHub from a gist or a repository. To share code using a gist, include the gist ID in the URL as follows:")
, indent (p (code (text " try.purescript.org?gist=<gist id>")))
, p (fold
[ text "The gist should contain PureScript module named "
, code (text "Main")
, text " in a file named "
, code (text "Main.purs")
, text " containing your PureScript code."
])
]
where
fromExample { title, source } =
link ("https://github.com/purescript/trypurescript/master/client/examples/" <> source) (text title)
examples =
[ { title: "Algebraic Data Types"
, source: "ADTs.purs"
}
, { title: "Loops"
, source: "Loops.purs"
}
, { title: "Operators"
, source: "Operators.purs"
}
]
The below is compiled using PureScript:

Metrics:
Adoption: PureScript is popular among functional programming enthusiasts, particularly in industries that require strong typing for safety and maintainability.
GitHub Stars: 7.5k+
Community: Though smaller than Flow, PureScript has a dedicated community focused on functional programming within JavaScript. It’s also gaining interest in projects requiring complex logic and mathematical computations.
Conclusion:
Flow and PureScript offer advanced capabilities for JavaScript developers, focusing on optimization, performance, and type safety. Closure Compiler excels at minification, dead code elimination, and optimizing large applications, while PureScript brings the power of functional programming and strong typing to the JavaScript ecosystem. Together, these compilers provide unique solutions for developers aiming to enhance the performance, maintainability, and scalability of their JavaScript applications.
Comentarios