In today's app-driven world, delivering a seamless and interactive user experience is crucial. But behind every polished interface is a well-structured design pattern that ensures smooth data flow, maintainable code, and easy testing. One of the most powerful patterns for creating responsive, data-rich applications is Model-View-ViewModel (MVVM). Whether you’re building for mobile, desktop, or even the web, MVVM offers a streamlined approach to managing the complex interactions between data and UI that keeps users engaged.
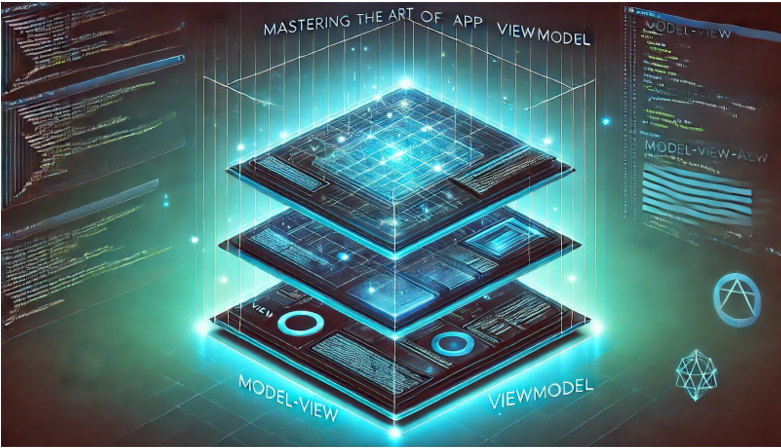
What Exactly is the Model-View-ViewModel (MVVM) Pattern?
MVVM is a design pattern that organizes the codebase into three distinct components: Model, View, and ViewModel. By separating an app’s functionality into these layers, MVVM creates a clear structure, reduces dependency issues, and enables smooth updates and testing.
Model – This is where the app’s data lives. It handles the logic for retrieving, storing, and managing data—often interacting with APIs, databases, or other external sources.
View – The View is the app’s user interface. It shows data to the user and takes input (like button clicks or form entries). Think of it as the presentation layer that users see.
ViewModel – The “go-between” that connects the Model and View. The ViewModel updates the View with new data from the Model and translates user actions into updates to the data in the Model. It also holds the app’s state, making sure that UI elements always display the most current data.
The MVVM pattern shines because it doesn’t let the Model or View interfere with each other directly; instead, they communicate through the ViewModel. This means if your data changes, the View updates seamlessly, and if the UI needs to change, your core data structure remains untouched.
Why MVVM? The Benefits That Keep Developers Coming Back
The MVVM pattern has several key benefits that make it a favorite among developers, especially for complex, interactive applications:
1. Two-Way Data Binding for a Dynamic UI
MVVM leverages two-way data binding, which means that when data in the Model changes, it instantly updates the UI. This creates a dynamic experience where users see data updates in real time without needing to refresh the page or app. This is ideal for apps like chat platforms, live sports scores, or stock trackers, where instant updates keep users engaged.
2. Testability and Clean Code
By separating business logic from the UI, MVVM makes testing much easier. Developers can test the ViewModel independently, which means fewer bugs sneak into the user interface. Each layer has a specific responsibility, reducing the chance of issues cascading throughout the app.
3. Modularity and Reusability
MVVM allows developers to reuse code and components, which is crucial for apps that scale over time. Since Views are decoupled from Models, you can change the UI without affecting data handling and vice versa. This makes it ideal for projects that need to be built quickly or updated frequently.
4. Improved Maintainability
With MVVM, it’s easier to track down issues, refactor code, or add new features. The clear division of responsibilities means that if you need to update the data layer (Model), the UI (View) can stay untouched. This separation of concerns is a lifesaver for teams, making the app easy to maintain, even as it grows.
MVVM Architecture :
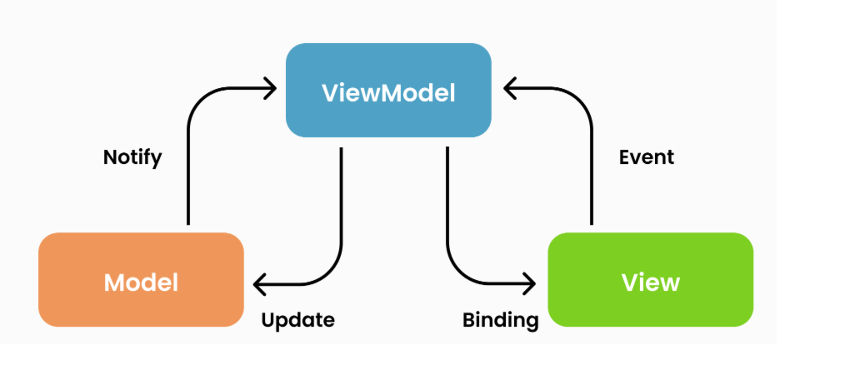
Real-World Examples: Where MVVM Thrives
Curious where MVVM really shines? Here are some real-world use cases and applications that harness the power of this design pattern:
1. Mobile Apps with Xamarin and SwiftUI
For mobile development, MVVM enables seamless updates to dynamic data and supports cross-platform development in frameworks like Xamarin and SwiftUI. Imagine a fitness tracking app where workout data updates instantly across the UI or a travel app where flight information is refreshed in real-time.
2. Modern Web Development with Angular and Vue.js
While Angular and Vue don’t strictly follow MVVM, they incorporate similar principles, particularly through the use of data binding and state management libraries like RxJS or Vuex. This makes it easier to manage complex state interactions in web apps, such as e-commerce sites, where shopping cart data updates instantly when users add items.
Breaking It Down: How MVVM Simplifies a Complex World
Consider an e-commerce app that tracks orders and shows user reviews. In an MVVM structure, this would look something like:
Model: The Model stores product details, order status, and reviews. It communicates with external APIs or databases for retrieving and updating this data.
View: The View displays product images, descriptions, and allows users to write reviews or track their order. This is the visible part of the app that users interact with.
ViewModel: The ViewModel acts as the middleman, handling commands from the user (like placing an order) and retrieving data to update the View. For instance, when a user submits a review, the ViewModel validates it and sends it to the Model. The Model updates its data, and the ViewModel instantly refreshes the View to show the new review.
With this setup, MVVM ensures that all layers work in harmony. The app is fast, responsive, and easy to test or update—no more tangled code slowing down development!
MVVM in Action: An Analogy to Illustrate
Imagine a news website that delivers live updates on breaking news. Here’s how MVVM would fit in:
Model: Acts like the newsroom database, storing all news articles, breaking news alerts, and video footage.
View: The public-facing website or app where readers view articles and breaking news alerts.
ViewModel: The editor, selecting and organizing the articles from the newsroom for the public, ensuring they see the latest stories without getting confused by backend details.
The editor (ViewModel) ensures readers (View) always have up-to-date news from the newsroom (Model), maintaining a smooth, efficient news delivery experience.
MVVM vs. MVC: Choosing the Right Design Pattern
While MVC (Model-View-Controller) and MVVM share similarities, there are key distinctions:
MVC: Works well for simple apps where the Controller directly handles user interactions, often suited for traditional web apps.
MVVM: Ideal for complex, interactive UIs and applications with two-way data binding, like desktop apps or mobile interfaces. MVVM’s ViewModel handles UI logic, making it easier to maintain and test.
In general, if your app relies on constantly updating data and has intricate UI components, MVVM is a strong choice. For simpler applications, MVC may be enough.
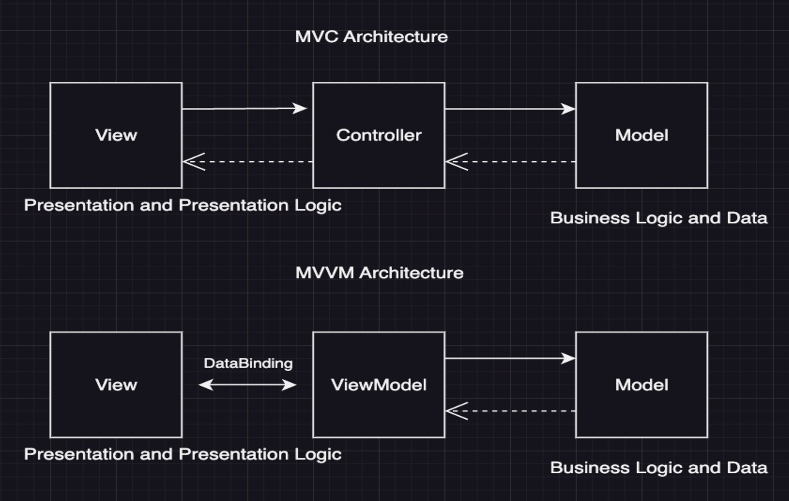
Tools and Frameworks that Support MVVM
Several popular tools support MVVM natively, making it easy to get started with this pattern:
Xamarin – Cross-platform mobile development framework that lets developers use MVVM across iOS and Android.
SwiftUI – Apple’s framework for iOS development embraces MVVM principles, especially with SwiftUI’s data binding capabilities.
Vue.js – Though not traditionally MVVM, Vue.js’s reactivity and state management support MVVM-like workflows, making it a go-to for web applications with real-time data.
Comments